How To Create A Website Using Python
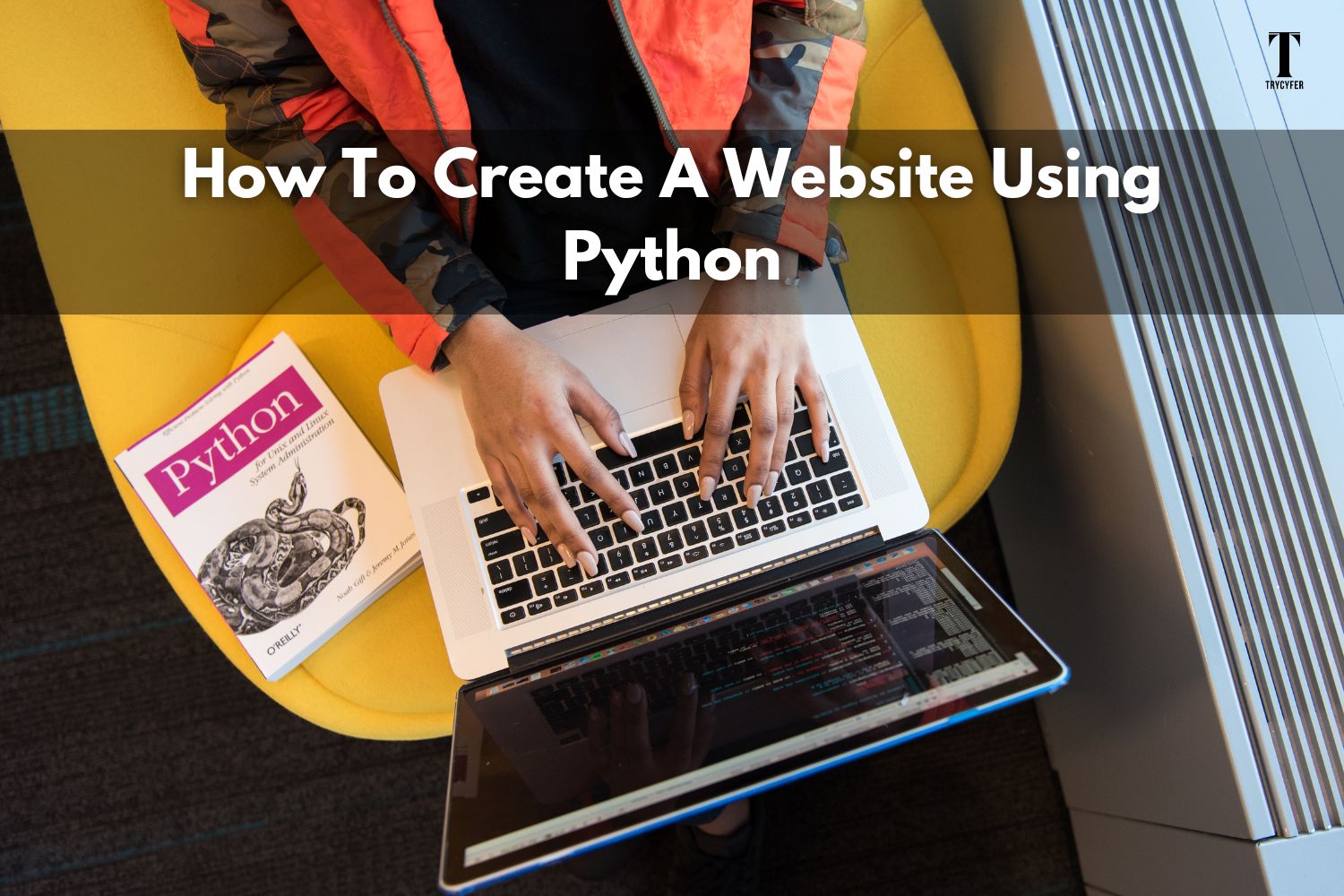
Developing a website combines creativity, technical skills, and problem-solving approach. Python, with its clarity and versatility, is an ideal choice for developing websites, whether you’re a beginner or an experienced developer. In this blog, we will explore the development of a website using Python, highlighting the essential tools, frameworks, and best practices.
It’s important to understand why Python is a popular choice for web development:
- Ease of Learning: Python is a beginner- friendly programming language and also has a clean syntax and readability.
- Versatility: Python is a general-purpose language, meaning it’s suitable for various applications, including web development.
- Extensive Libraries: Python has a vast range of libraries and frameworks, like Django and Flask, that streamline web development tasks.
- Community Support: Python has a vast community that remains active and offers plenty of resources, tutorials, and tools.
Step 1: Setting Up the Development Environment
Setting up the development environment is the foundational step in creating a website using Python. Before you start coding, you’ll need to set up your development environment to create a website. This involves installing Python, a code editor, and necessary libraries. Here’s an explanation of how to set up your environment:
- Install Python: Download and install the latest version of Python from the official Python website (https://www.python.org/downloads/). Ensure you add Python to your system’s PATH during installation.
- Choose a Code Editor: Select a code editor that suits your needs. Popular choices include Visual Studio Code, PyCharm, and Sublime Text. These editors offer syntax highlighting, debugging tools, and other features that make coding easier.
- Set Up a Virtual Environment: A virtual environment is a tool that helps keep dependencies required by different projects in separate places by creating isolated Python environments. You can make a virtual environment by running the following commands:
pip install virtualenv
virtualenv myprojectenv
- Activate the Virtual Environment:
On Windows: myprojectenv\Scripts\activate
On Mac/Linux: source myprojectenv/bin/activate
Step 2: Choose a Web Framework
Choosing the proper web framework is important in developing your website using Python. Python offers several frameworks for web development, but the most popular ones are Django and Flask. Both have their strengths and are suited for different types of projects.
Django
Django is a high-level Python web framework offering rapid development and clean, pragmatic design. It’s well-suited for larger projects and comes with many built-in features, such as an admin interface, authentication, and an ORM (Object-Relational Mapping) system.
Advantages of Django:
- Batteries Included: Django includes most of the features you need to develop a web application out of the box.
- Security: Django’s robust built-in security features, including protection against SQL injection, cross-site scripting, and clickjacking, provide a strong foundation for your web applications, giving you confidence in their safety.
- Scalability: Django is designed to handle high traffic and large amounts of data.
When to Use Django:
- If you’re building a complex application with many components.
- If you need a framework that provides many built-in tools and features.
Flask
Flask is a compact web framework for Python. Its compact framework allows you to choose the components you want to use. Flask is perfect for small to medium-sized applications.
Advantages of Flask:
- Flexibility: Flask is very flexible and doesn’t impose any dependencies on external libraries or tools.
- Extensibility: You can easily extend Flask, which has numerous extensions for different functionalities.
- Simplicity: Flask has a smaller codebase, making it easier to understand and work with.
When to Use Flask:
- If you’re building a small or straightforward application.
- If you want more control over the components of your web application.
Step 3: Create a Basic Web Application
Creating a basic web application is important in understanding how to build and structure a website using Python. This step involves setting up the initial project structure, defining the main components, and running your application to ensure everything works correctly. Once you’ve chosen a framework, it’s time to create a basic web application. We’ll provide examples using both Django and Flask.
Using Django
1. Install Django:
pip install django
2. Create a Django Project:Â
django-admin startproject mywebsite
cd mywebsite
3. Start the Development Server:
python manage.py runserver
Open your browser and navigate to http://127.0.0.1:8000/. You should see the Django welcome page.
4. Create a Django App:
Django projects are divided into apps, which are modules that contain models, views, and controllers. Create a new app using:
python manage.py startapp myapp
5. Define Models:
Models define the structure of your database. In myapp/models.py, define your models:
from django.db import models
class Post(models.Model):
    title = models.CharField(max_length=200)
    content = models.TextField()
    published_date = models.DateTimeField(auto_now_add=True)
6. Create Views:
Views handle the logic of your application. In myapp/views.py:
from django.shortcuts import render
from .models import Post
def home(request):
    posts = Post.objects.all()
    return render(request, ‘home.html’, {‘posts’: posts})
7. Create Templates:
Templates are HTML files that define how your content is displayed. In myapp/templates/home.html:
<!DOCTYPE html>
<html>
<head>
    <title>My Website</title>
</head>
<body>
    <h1>My Blog</h1>
    {% for post in posts %}
        <h2>{{ post.title }}</h2>
        <p>{{ post.content }}</p>
        <p>Published on {{ post.published_date }}</p>
    {% endfor %}
</body>
</html>
8. Configure URLs:
Define the URL patterns in mywebsite/urls.py:
from django.contrib import admin
from django.urls import path
from myapp import views
urlpatterns = [
    path(‘admin/’, admin.site.urls),
    path(”, views.home, name=’home’),
]
9. Run Migrations:
Apply the database migrations to create the tables:
python manage.py makemigrations
python manage.py migrate
10. View the Result:
Run the development server and view your website at http://127.0.0.1:8000/.
Using Flask
1. Install Flask:
pip install flask
2. Create a Flask App:
Create a file named app.py and add the following code:
from flask import Flask, render_template
app = Flask(__name__)
@app.route(‘/’)
def home():
    posts = [
        {“title”: “First Post”, “content”: “This is the first post.”},
        {“title”: “Second Post”, “content”: “This is the second post.”}
    ]
    return render_template(‘home.html’, posts=posts)
if __name__ == ‘__main__’:
    app.run(debug=True)
3. Create Templates:
Create a directory named templates and add a file named home.html:
<!DOCTYPE html>
<html>
<head>
    <title>My Website</title>
</head>
<body>
    <h1>My Blog</h1>
    {% for post in posts %}
        <h2>{{ post.title }}</h2>
        <p>{{ post.content }}</p>
    {% endfor %}
</body>
</html>
4. Run the Flask App:
python app.py
Open your browser and navigate to http://127.0.0.1:5000/ to view your website.
Step 4: Adding Functionality
After setting up your web application’s basic structure, the next step is to add functionality. It involves creating dynamic features that allow users to interact with your application, process inputs, and deliver appropriate responses. This step can vary depending on whether you use Django or Flask, but the basic principles will remain similar.Â
1. Forms and User Input:
Django: Use Django’s forms framework to handle user input securely and easily.
Flask: Flask-WTF is an extension that integrates Flask with WTForms, allowing you to create forms and handle user input.
2. User Authentication:
Django: Django provides built-in authentication, including user registration, login, and logout.
Flask: Flask-Login is an extension that adds user session management to your Flask application.
3. Database Integration:
Django: Django’s ORM makes it easy to interact with databases without writing SQL.
Flask: SQLAlchemy is a popular ORM for Flask that simplifies database operations.
4. API Integration:
Django: Django REST framework is a powerful toolkit for building Web APIs.
Flask: Flask-RESTful is an extension for building REST APIs with Flask.
Step 5: Testing and Debugging
Once the website’s functionality has been added, the next important step is testing and debugging. This step ensures that your website works as planned and is free from errors or issues that could affect user experience or security. Testing and debugging are critical for delivering a reliable and high-quality product.
- Run Tests: Conduct unit and integration tests to ensure all parts of your web application work correctly.
- Identify Issues: Look for bugs or errors that may cause the application to malfunction.
- Use Debugging Tools: Utilize debugging tools to track down and fix issues in the code.
- Cross-Browser Testing: Test your website across different browsers and devices to ensure compatibility.
- Optimize Performance: Identify and resolve performance bottlenecks to improve speed and efficiency.
- User Testing: Have real users test the application to gather feedback and spot any overlooked issues.
- Final Review: Perform a thorough final check to ensure the application is ready for deployment.
Step 6: Deploying Your Website
After thoroughly testing and debugging your web application, it’s time to make it live for users. Deploying your website involves moving your code from a development environment (like your local machine) to a production environment where anyone on the internet can access it.
- Choose a Hosting Service: Select a reliable hosting provider to store your website files and make your site accessible online.
- Set Up a Domain Name: Register a domain name that reflects your brand or project, making it easy for users to find your site.
- Configure Server Settings: Adjust server settings to ensure optimal performance, security, and compatibility with your application.
- Upload Files: Transfer your website files from your local development environment to the hosting server using tools like FTP.
- Set Up a Database: If your site requires one, configure a database on the server to manage and store data efficiently.
- Perform Final Tests: Test the website in its live environment to ensure everything works smoothly and as expected.
- Monitor Performance: Keep an eye on your website’s performance and security post-deployment, making necessary adjustments as needed.
Conclusion
Deploying a Python-based website involves critical steps that ensure your web application is ready for public access. From choosing the hosting provider to configuring the server, deploying the code, and ongoing maintenance, each phase is crucial to the success of your project.